In this project we measure the energy produced by a solar Heater; we use a microcontroller, three temperature sensors and a flow sensor. The microcontroller made the calculations for the energy integration and the calculation of volume of water consumed. We send this data to datadrop, for its manipulation and visualization.
datos2 = Dataset[Databin["mCC3DAXt"]];
data = datos2[
All, {"tempTank" -> N[Quantity[#tempTank/10, "DegreesCelsius"]] &,
"tempIn" -> N[Quantity[#tempIn/10, "DegreesCelsius"]] &,
"tempOut" -> N[Quantity[#tempOut/10, "DegreesCelsius"]] &,
"ms" -> Identity[#ms] &,
"Volume" -> N[Quantity[#Volume/100, "liters"]] &,
"Energy" -> N[Quantity[#Energy/100, "Kilojoules"]] &,
"id" -> Identity[#id] &, "Timestamp" -> Identity[#Timestamp] &}][
All, Association];
dataSort = data[Reverse, Reverse]
We totalize variables such as energy and volume of water consumed during the life of the Arduino. So we initialize all the variables we need to show on the sketch.
fVol[n_] := n/100
ftemp[n_] := n/10
fCel[n_] := N[Quantity[n, "DegreesCelsius"]]
fVol1[n_] := N[Quantity[n, "Liters"]]
fEngy[n_] := N[Quantity[n, "Kilojoules"]]
datos3 = datos2[All,
{
"tempTank" -> ftemp,
"tempIn" -> ftemp,
"tempOut" -> ftemp,
"Volume" -> fVol,
"Energy" -> fVol
}
]
datos4 = datos3[All, {
"tempTank" -> fCel,
"tempIn" -> fCel,
"tempOut" -> fCel,
"Volume" -> fVol1,
"Energy" -> fEngy
}
]
es = TimeSeries[Databin["mCC3DAXt"]];
time = DateObject /@ Flatten[es[[1]][[2, 2]]];
en1 = fVol /@ Flatten[es[[1]][[2, 1]]];
en2 = fEngy /@ en1;
tsEngy = TimeSeries[en2, {time}];
vol1 = fVol /@ Flatten[es[[7]][[2, 1]]];
vol2 = fVol1 /@ vol1;
tsVol = TimeSeries[vol2, {time}];
temptank = ftemp /@ Flatten[es[[6]][[2, 1]]];
temptank1 = fCel /@ temptank;
tstempTank = TimeSeries[temptank1, {time}];
tempIn = ftemp /@ Flatten[es[[4]][[2, 1]]];
tempIn1 = fCel /@ tempIn;
tstempIn = TimeSeries[tempIn1, {time}];
tempOut = ftemp /@ Flatten[es[[5]][[2, 1]]];
tempOut1 = fCel /@ tempOut;
tstempOut = TimeSeries[tempOut1, {time}];
totalVolume1 = Total[vol2];
totalEnergy1 = Total[en2];
Including the starting time of data and the end time.
lastday = DateList[ Last[Flatten[tstempTank[[2, 2]]]]][[3]]
firstday = DateList[ First[Flatten[tstempTank[[2, 2]]]]][[3]];
firstmonth = DateList[ First[Flatten[tstempTank[[2, 2]]]]][[2]];
lastmonth = DateList[ Last[Flatten[tstempTank[[2, 2]]]]][[2]];
dates = tstempTank["Dates"];
So at the end we can visualize the data on a dice Interface.
Manipulate[
DynamicModule[{firstPage, stadistics},
firstPage = Show[GraphicsGrid[{
{AngularGauge[totalVolume1, {0, 3000},
ScaleOrigin -> {6 Pi/5, -Pi/5}, PlotTheme -> "Business",
GaugeLabels -> "Value", PlotLabel -> "Volume L",
ImageSize -> 300],
Animate[
Graphics3D[{Red,
Rotate[First@PolyhedronData["Spikey"],
p*2 \[Pi], {1, 1, 1}]},
Boxed -> False, AspectRatio -> 1, ImageSize -> Medium],
{p, 0, Infinity}, Paneled -> False,
AnimationRunning -> False
]
,
Row@{ThermometerGauge[Last[tempIn1], {0, 120},
PlotLabel -> "Temperature In", TargetUnits -> "Celsius",
GaugeLabels -> Full, PlotTheme -> "Marketing",
ImageSize -> {100, 300}],
ThermometerGauge[Last[tempOut1], {0, 120},
PlotLabel -> "Temperature Out", TargetUnits -> "Celsius",
GaugeLabels -> Full, PlotTheme -> "Marketing",
ImageSize -> {100, 300}]}},
{AngularGauge[totalEnergy1, {0, 5000},
ScaleOrigin -> {6 Pi/5, -Pi/5}, PlotTheme -> "Business",
GaugeLabels -> "Value", PlotLabel -> "Energy kJ",
ImageSize -> 300],
SpanFromAbove,
ThermometerGauge[Last[temptank1], {0, 120},
PlotLabel -> "Tank Temperature", TargetUnits -> "Celsius",
GaugeLabels -> Full, PlotTheme -> "Marketing",
ImageSize -> {200, 300}]}}
,
Frame -> False,
Alignment -> Center,
Background -> Transparent,
BaseStyle -> {FontColor -> GrayLevel[0.6], FontFamily -> "Times",
30}
]];
stadistics =
Show[GraphicsGrid[{{relojes, SpanFromLeft, SpanFromLeft},
{With[{dataAssoc = GroupBy[dates, #["Month"] &]},
Manipulate[Manipulate[
DateListPlot[
TimeSeriesWindow[tsEngy,
DateObject[{2017, month, day}, "Day"]],
PlotTheme -> "Web",
Joined -> False,
Filling -> Axis,
ImageSize -> 200,
PlotRange -> All,
PlotLabel -> DateObject[{2017, month, day}, "Day"]
],
Style["Energy Produced", 25, Bold, FontFamily -> "Times",
TextAlignment -> Center],
{day,
Sequence @@ (MinMax[#["Day"] & /@ (dataAssoc[month])]), 1},
Paneled -> False],
{{month, lastmonth},
Sequence @@
MinMax[Keys[MinMax[#["Day"] & /@ #] & /@ dataAssoc]], 1}
, SynchronousUpdating -> True, Paneled -> False]],
With[{dataAssoc = GroupBy[dates, #["Month"] &]},
Manipulate[Manipulate[
DateListPlot[
TimeSeriesWindow[tstempTank,
DateObject[{2017, month, day}, "Day"]],
PlotTheme -> "Web",
Joined -> False,
Filling -> Axis,
ImageSize -> 200,
PlotRange -> All,
PlotLabel -> DateObject[{2017, month, day}, "Day"]
],
Style["Tank Temperature", 25, Bold, FontFamily -> "Times",
TextAlignment -> Center],
{day,
Sequence @@ (MinMax[#["Day"] & /@ (dataAssoc[month])]), 1},
Paneled -> False],
{{month, lastmonth},
Sequence @@
MinMax[Keys[MinMax[#["Day"] & /@ #] & /@ dataAssoc]], 1},
SynchronousUpdating -> True, Paneled -> False]],
With[{dataAssoc = GroupBy[dates, #["Month"] &]},
Manipulate[Manipulate[
DateListPlot[
TimeSeriesWindow[tsVol,
DateObject[{2017, month, day}, "Day"]],
PlotTheme -> "Web",
Joined -> False,
Filling -> Axis,
ImageSize -> 200,
PlotRange -> All,
PlotLabel -> DateObject[{2017, month, day}, "Day"]
],
Style["Hot Water Consumed ", 25, Bold,
FontFamily -> "Times", TextAlignment -> Center],
{day,
Sequence @@ (MinMax[#["Day"] & /@ (dataAssoc[month])]), 1},
Paneled -> False],
{{month, lastmonth},
Sequence @@
MinMax[Keys[MinMax[#["Day"] & /@ #] & /@ dataAssoc]], 1},
SynchronousUpdating -> True, Paneled -> False]]}},
ItemAspectRatio -> 1 ,
ImageSize -> 1000,
Frame -> False,
Alignment -> Center,
Background -> Transparent,
BaseStyle -> {FontColor -> Black, FontFamily -> "Times", 30}]
];
Show[Switch[vizualization, 1, firstPage, 2, stadistics],
ImageSize -> {1000, 700}]
],
Control[{{vizualization, 1, ""}, {1 -> "Parameters",
2 -> "Statistics"}, Setter}],
PaneSelector[{
1 -> Column@{Spacer@2,
Style["Measurement and Visualization of Energy production \
\"Solar Heaters\"", 40, Bold, FontFamily -> Times,
TextAlignment -> Center]},
2 -> Column@{Spacer@2,
Style["Statistics", 40, Bold, FontFamily -> Times,
TextAlignment -> Center]}},
Dynamic@vizualization],
SaveDefinitions -> True,
Paneled -> False]
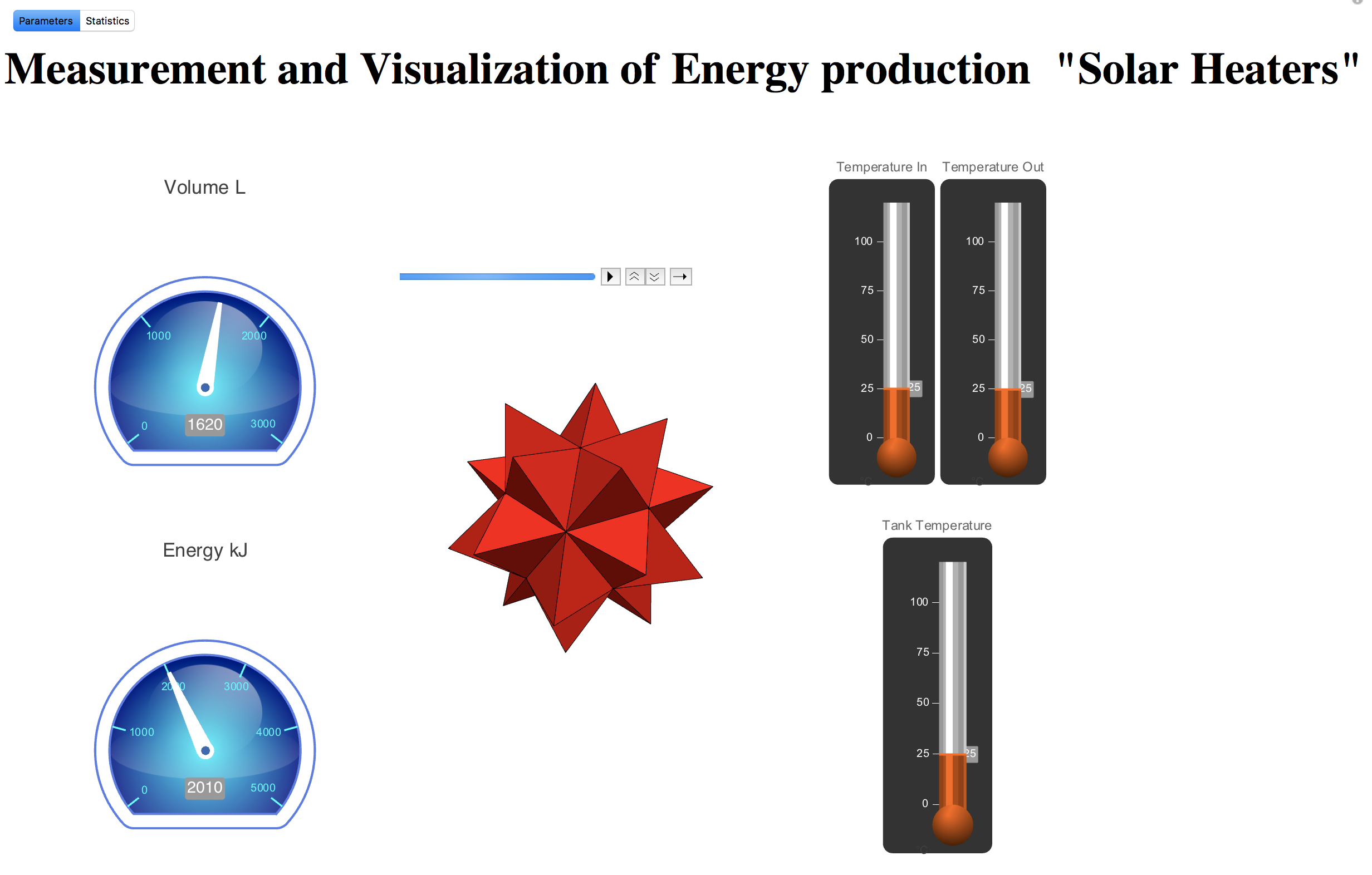
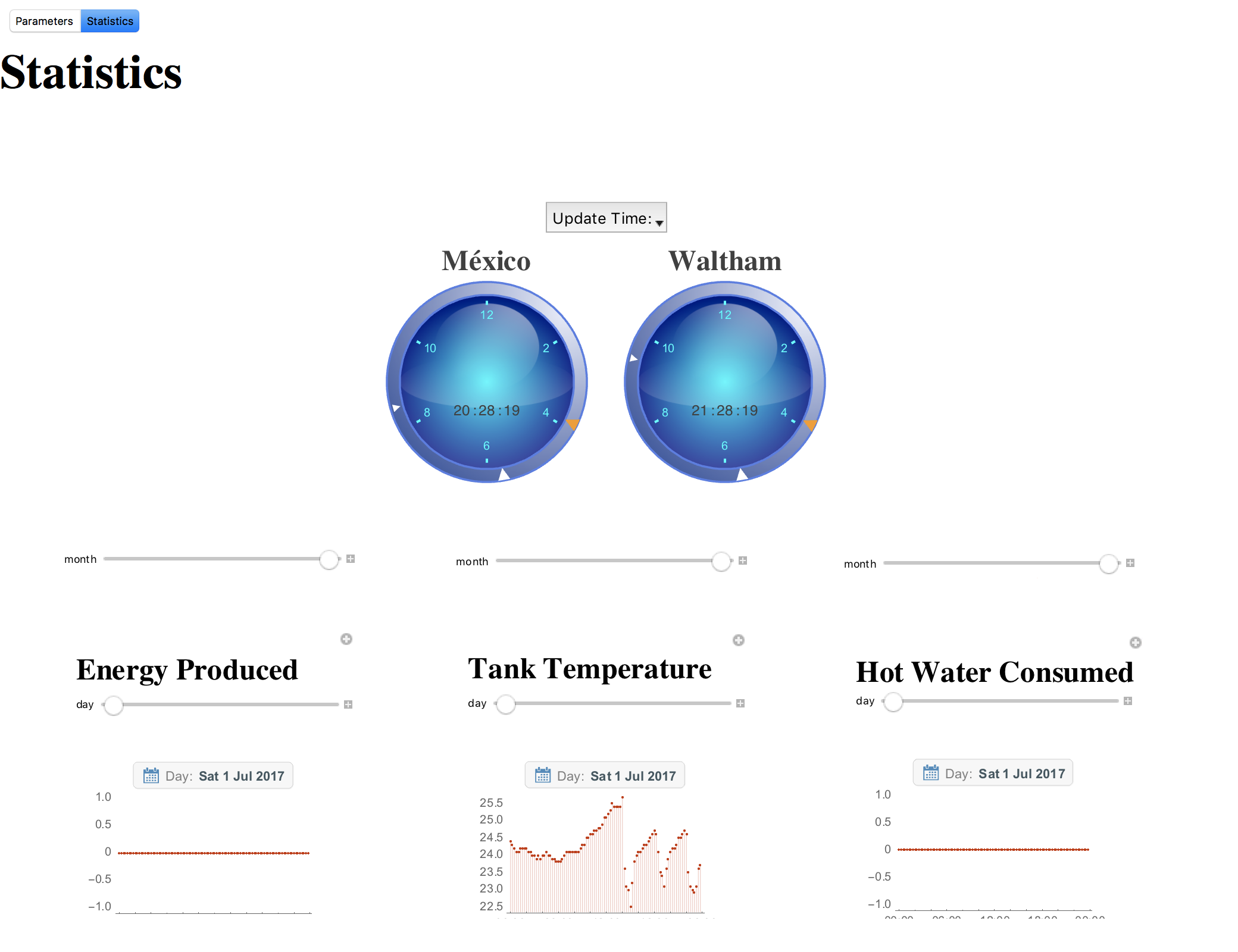