Hello, I have a question about selecting items in a list that match any item in another list.
Here is the problem. I'm having 2 lists named, list and sum respectively.
list = {3, 2, 1, 6, 5, 4, 9, 7}
sums = {5, 6, 9, 10}
How to I find pairs of numbers in list that's matching any of the numbers in sums?
To start off, i generated possible number of pairs from list, using IntegerPartition command that will use only numbers from list to generate pairs.
(# -> IntegerPartitions[#, {2}, list]) & /@ sums // Column
5->{{4,1},{2,3}}
6->{{4,2},{5,1},{3,3}}
9->{{7,2},{4,5},{6,3}}
10->{{7,3},{9,1},{4,6},{5,5}}
In the above output, sums list of {5,6,9,10} can be written in pairs using the numbers in the list {3, 2, 1, 6, 5, 4, 9, 7}.
Transforming my list into possible pairs:
Partition[#, {2}] & /@ Permutations[list]
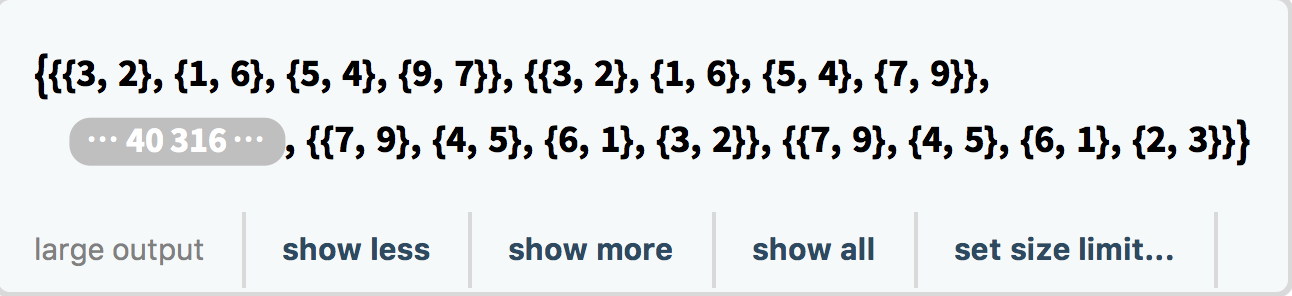
So, there are 40,320 combinations, out of these, how can I select a list that has pairs which sum up to any of the numbers in the sums list?