I recently had a job interview where I was given the following problem and I decided to tackle it with Mathematica vs. Groovy; it seemed very simple to accomplish using Mathematica: (Hopefully they take kindly to it)
- Theres a fake REST service available for testing at: http://jsonplaceholder.typicode.com/
- Write some code in the Groovy programming language that uses the
posts API published at http://jsonplaceholder.typicode.com/posts,
which reports back some fake blog posts including a userid, post id, title, and body.
- Retrieve the records from the API and generate a report that lists only the users who wrote posts where the post title starts with the letter s along with the number of such posts for each of those users.
- Use the user's name in the report, not the numerical user ID.
- Return to us the report that's generated, along with the (working) code that generated it.
First, let's import our posts from the website as RawJSON format and generate a Dataset from it which is much more convenient to work with:
posts = Import["http://jsonplaceholder.typicode.com/posts", "RawJSON"];
postsDataSet = Dataset[posts]
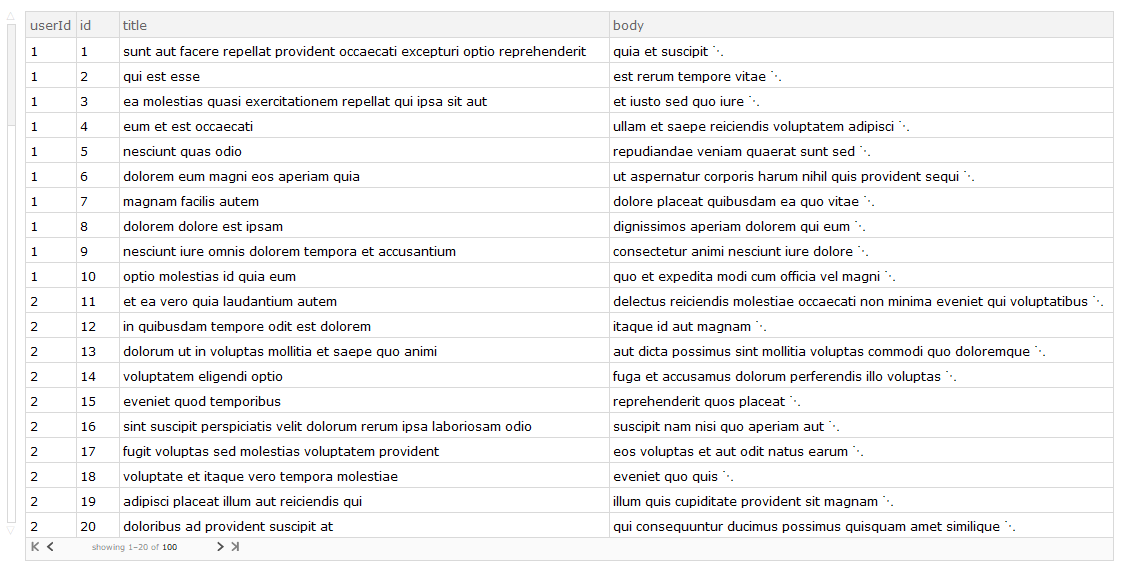
Next, let us filter out all of the posts whose bodies of text start with the string character "s":
filterPostsDataSet = postsDataSet[Select[StringTake[#title, 1] == "s" &]]
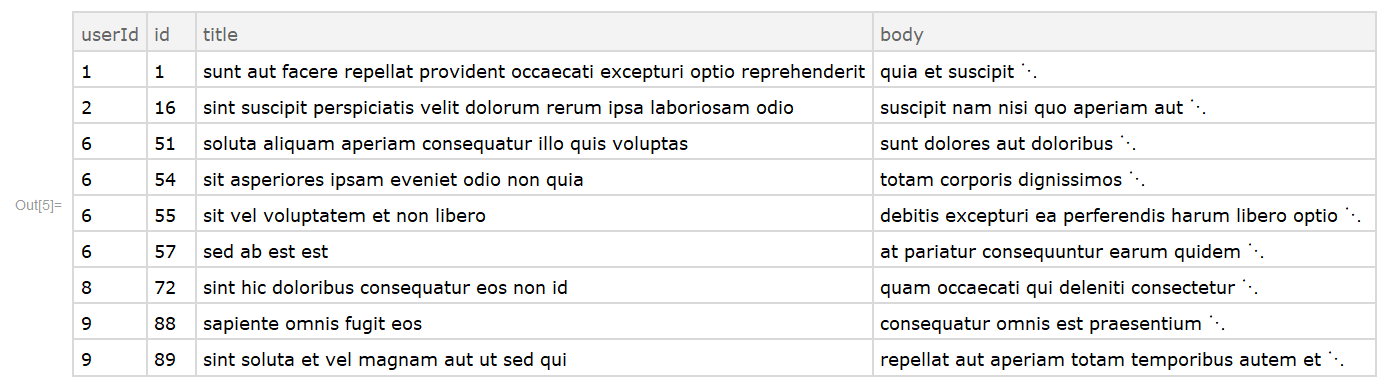
We are left with 9 entries and all we need to do is keep the userId values associated with these:
userIDs = filterPostsDataSet[All, "userId"]

Now we need to retrieve the user's information attached to these Id values; although not specifically stated I assumed these could be found at http://jsonplaceholder.typicode.com/users. Thus, we simply import these values and, like before, wrap them in a Dataset.
users = Import["http://jsonplaceholder.typicode.com/users", "RawJSON"];
usersDataSet = Dataset[users]
Although I feel there is a more elegant way - I am going to make a list of just the users names from this Dataset to work with using the following:
userResults =
Table[usersDataSet[Select[#id == userIDs[[i]] &]][[1]]["name"], {i, 1, Length[userIDs]}]
Additionally, I want to keep a list of the unique user names since some might be duplicated in the previous list:
uniqueUsers = DeleteDuplicates[userResults]
Finally, I will create a final list which is essentially my required report, which will be a list of pairs. Each pair will contain the unique user name as well as the count of how many times that unique user name appeared in the original list. I put this in TableForm just for aesthetic value. This can then be exported to the desired format later on if necessary.
uniqueUserPosts = Table[{uniqueUsers[[i]], Count[userResults, uniqueUsers[[i]]]}, {i, 1, Length[uniqueUsers]}] // TableForm
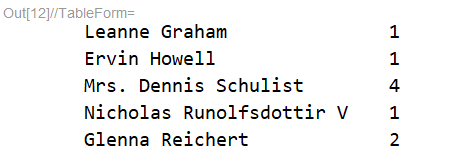
In conclusion, I think that in the future using Mathematica in working with REST API's could and should be allowed if not encouraged as a proper scripting languages in many commercial and industrial settings. So - what do you guys think?? Is this better or worse than what could be done in Groovy or other scripting languages? Also, I feel that I could condense and optimize my code in areas and would love to hear feedback on that.