Description:
This work is the search for scalene SEPP (Square Even-Prime-Prime) triangles with integer area. They're semi-rare to be found. There are no square odd-prime-prime triangles with integer area, as there are no triangles with the three odd sides and integer area. Its first representative is the triangle with sides 3-4-5. Below is the representative image of a SEPP:
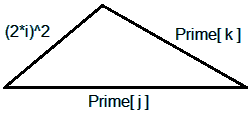
I've elaborated this simple code below to find the triangles with these properties. I opted to use parallel computing (8 kernels) and measured the computing time of each evaluate. The sides have the measurement until just below the quantity required in "n" (< n). The answer is in the form: "Quantity used" {"side a", "side b", "side c"} {area} graphics, with absolute time just below.
Objective and Coding:
The main objective here is to find how many of these exist for sides varying up to 10,100, 1000
in amounts of powers of 10 (10^x). Following just one example with the sides up to 50 to test the code; only 1 triangle found, the 3-4-5:
Parallelize[n = 50;
p = PrimePi[n];
Do[a = (2*i)^2; b = Prime[j]; c = Prime[k];
If[c < a + b \[And] a < b + c \[And] b < a + c \[And]
Area[SSSTriangle[a, b, c]] \[Element] Integers,
Print[n, {a, b, c}, {Area[SSSTriangle[a, b, c]]},
Graphics[SSSTriangle[a, b, c]]]], {i, 1,
IntegerPart[Sqrt[n - 1]/2]}, {j, 2, p - 1}, {k, j + 1,
p}]] // AbsoluteTiming
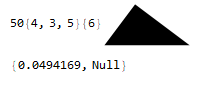
Now a code modification to find multiple results of the processing time in just one evaluation. The example below is programmed to calculate from 10 to 100 with steps of 10 {m,10,100,10}:
Do[Print[Parallelize[n = m;
p = PrimePi[n];
Do[a = (2*i)^2; b = Prime[j]; c = Prime[k];
If[c < a + b \[And] a < b + c \[And] b < a + c \[And]
Area[SSSTriangle[a, b, c]] \[Element] Integers, Null], {i, 1,
IntegerPart[Sqrt[n - 1]/2]}, {j, 2, p - 1}, {k, j + 1, p}]] //
AbsoluteTiming], {m, 10, 100, 10}]
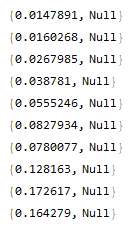
Above you can change in the code the part {m,10,100,10} by {m,{100,140,210}} to find, for example, the result for specific quantities of 100, 140, 210 etc. You can also change the Null part in the code by Print[n,{a,b,c},{Area[SSSTriangle[a,b,c]]},Graphics[SSSTriangle[a,b,c]]] to have multiple responses seeking the triangles.
Calculation and Results:
To carry out the evaluation in this work I used the following machine (only to have an idea of the processing used):
Intel(R) Core(TM) i7-9700K CPU @ 3.60GHz, 3600 Mhz, 8 Core(s), 8 Logic Processor(s) (run with 8 Kernels), RAM 16.0 GB, BaseBoard B360M AORUS Gaming 3, X64, NVIDIA GeForce GTX 1060 6GB.
The following table was assembled with the data of the quantities (maximum values for the side) in "n" and the absolute times (seconds) spent on parallel computing:
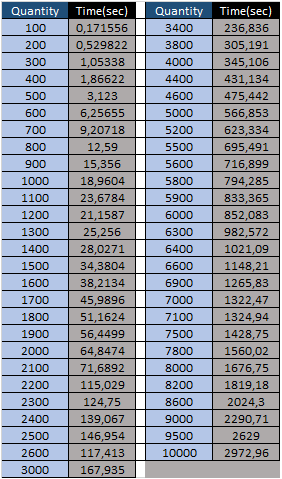
Now the results found using values with powers of 10:
- From values up to 10 (<10) and up to 100 (<100):
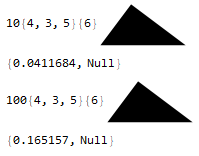
- The result for sides up to 1000 (<1000):
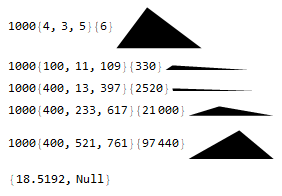
- And finally the result for sides up to 10000 (<10000):
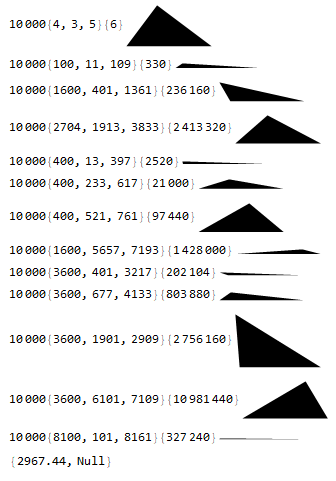
Time Prediction (Fitting Model):
I also made an attempt to predict the time required to calculate and find triangles with sides larger than 10000, so I used FindFit as follows (I did using "a.x^b" and "a.x^b.c^x"). I chose reduce the x-axis in a factor of 10 to make the fit (do not know how this affected the accuracy or if has affected..?), below is the example of the first fit (result with the fit of the data from 1000 to 10000 with steps of 1000 and with the prediction for 10^5):
y = a*x^b // StandardForm
data = {{10, 0.171556}, {20, 0.529822}, {30, 1.05338}, {40,
1.86622}, {50, 3.123}, {60, 6.25655}, {70, 9.20718}, {80,
12.59}, {90, 15.356}, {100, 18.9604}, {110, 23.6784}, {120,
21.1587}, {130, 25.256}, {140, 28.0271}, {150, 34.3804}, {160,
38.2134}, {170, 45.9896}, {180, 51.1624}, {190, 56.4499}, {200,
64.8474}, {210, 71.6892}, {220, 115.029}, {230, 124.75}, {240,
139.067}, {250, 146.954}, {260, 117.413}, {300, 167.935}, {340,
236.836}, {380, 305.191}, {400, 345.106}, {440, 431.134}, {460,
475.442}, {500, 566.853}, {520, 623.334}, {550, 695.491}, {560,
716.899}, {580, 794.285}, {590, 833.365}, {600, 852.083}, {630,
982.572}, {640, 1021.09}, {660, 1148.21}, {690, 1265.83}, {700,
1322.47}, {710, 1324.94}, {750, 1428.75}, {780, 1560.02}, {800,
1676.75}, {820, 1819.18}, {860, 2024.3}, {900, 2290.71}, {950,
2629}, {1000, 2972.96}};
FindFit[%, a*x^b, {a, b}, x]
Table[a*x^b /. %, {x, 100, 1000, 100}] \[And]
Table[a*x^b /. %, {x, {10000}}]
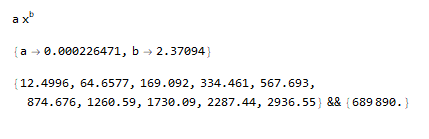
This chart was created using the real absolute time data as well as the two curves generated by FindFit that I tested:
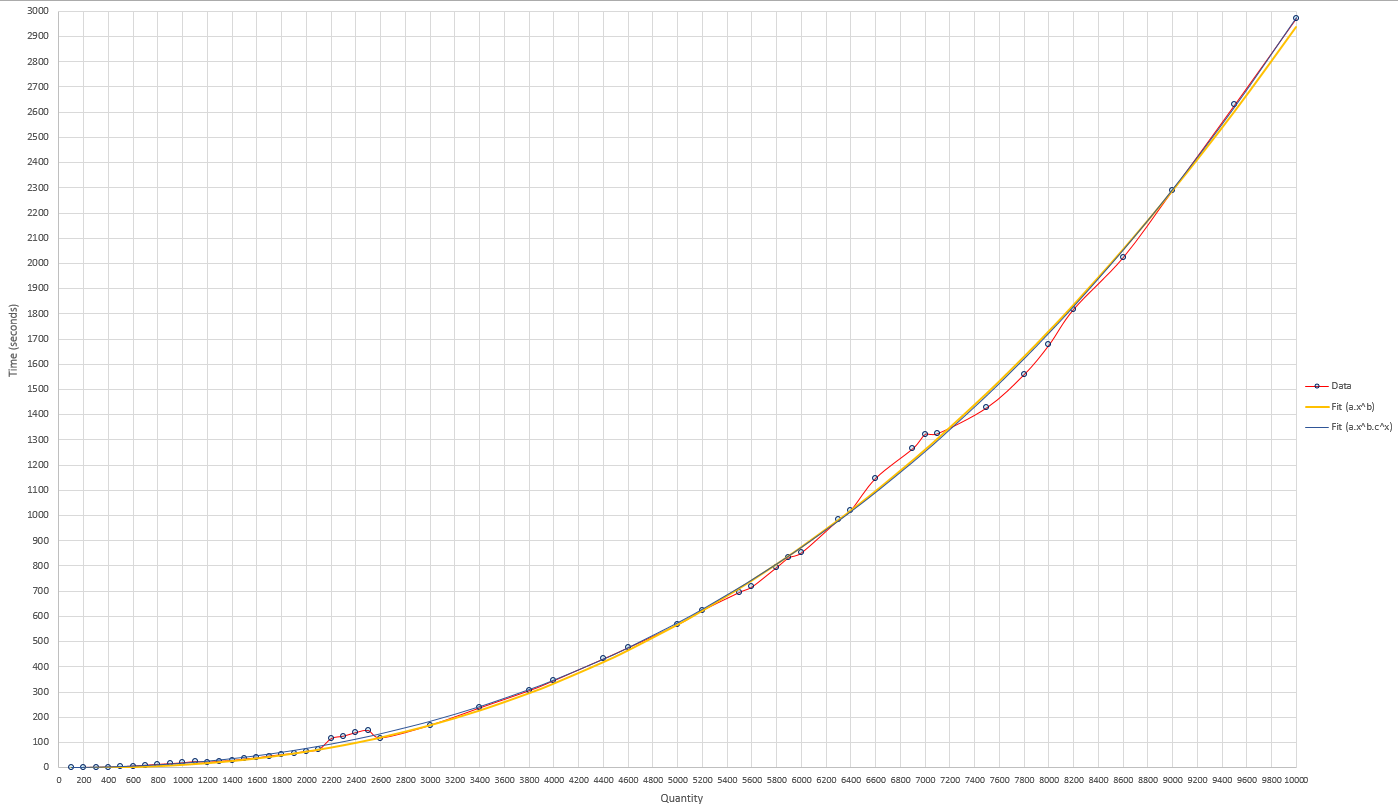
Conclusion:
There are only 13 scalene SEPP triangles with integer area and sides varying up to 10000 (10^4).
The curves used in FindFit gave very divergent values to predict the time required to evaluate with the sides up to 100000 (10^5), and the curve fit "a.x^b" (fit 1) was more optimistic and estimated that it would take 8 days of computation in parallel, while the curve fit "a.x^b.c^x" (fit 2) estimated it would take 171.5 days! ... Anyway are very long computing time to calculate all the possibilities of sides up to 10^5.
To choose the best fit curve to be able to predict with longer times, I evaluated with the sides up to 15000 to have a real point of time and get to know which curve approaches better. The real time for sides up to 15000 was 7428.57 seconds. The fit 1 curve came closest to the value with a prediction of 7679.62 seconds, while the fit 2 curve estimated a time of 8452.19 seconds. The "fit 1" curve had a difference of 4 minutes and 11 seconds or approximately 3.4% of the real value.
A Few Questions (that I have):
Is there any way to make these codes faster or more efficient? Any other way to find that kind of triangle using codes?
Is there a better way to use FindFit in this case to have a more accurate prediction? Maybe another function or more/less data? How to know the correct function model?
How many of these triangles will there be if we search for sides up to 10^5 or even 10^6? Would anyone have any idea to help me find it?
Thank you very much to everyone in the community.