Thanks Daniel Lichtblau for the suggestion to submit the function to the W. F. R.!
Thanks J. M. for the suggestion to expand the list of vitamins available for this function.
Thanks Wolfram Function Repository team for approving the VitaminData function.
The VitaminData function has been improved and expanded to be used more fully:
In this function there are 50 vitamins/vitamers organized into 20 vitamer groups. The function can provide the chemical and physical data of each molecule, as well as its 2D and 3D visualization, information on its chemical bonds and provide the atom counts with their respective charges. There are also 27 relative vitamin content properties among the 15 relative groups available to provide values for a specific food. The function is available to work with all 1816 food types from the Wolfram knowledgebase. This function can also generate nutritional information in the form of food labels for each of the foods. Finally, as a special option, you can also create food rankings for many types of vitamins (for all the relative vitamin content available).
New arguments and options have been added to the function.
The function can be used with the ResourceFunction["VitaminData"] command.
List of options for the function:
ResourceFunction["VitaminData"]["OptionalsList"]

Below is the option that shows the list of all vitamins/vitamers usable in the function (organized in their groups):
ResourceFunction["VitaminData"]["VitamersList"]
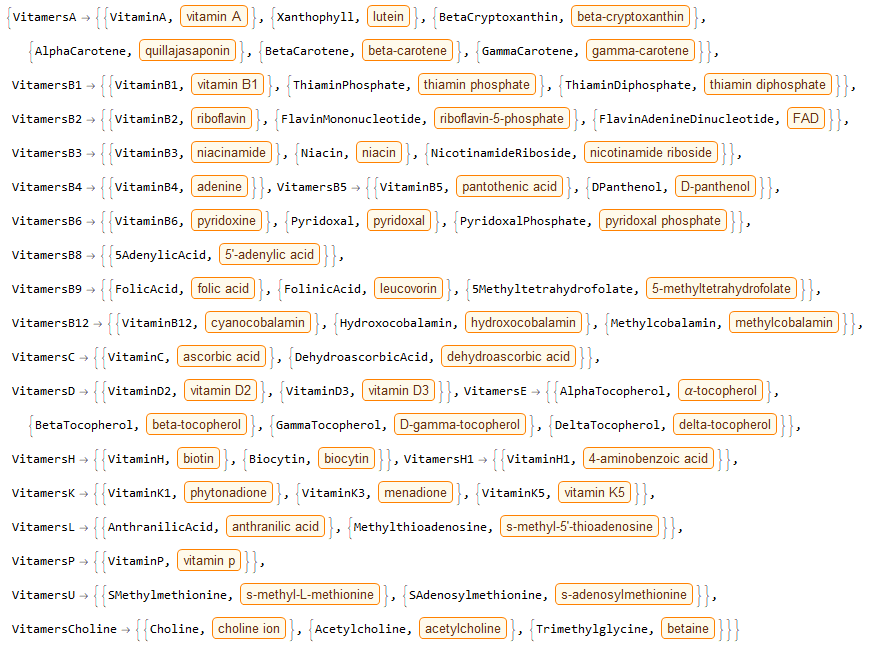
There are many other options and uses for the function, and anyone interested can take a look at the VitaminData function page:
https://resources.wolframcloud.com/FunctionRepository/resources/VitaminData
Thanks.