Ain't it the truth. When it comes to stuff like this retail non-professionals like myself play 5th fiddle when it comes to getting live data into the box to feed MMA apps. And I totally agree there is nothing on this planet remotely as good as Mathematica so we soldier on. But there are workarounds which don't involve a live feed into MMA. I trade option spread strategies at Schwab with TradingView and ThinkOrSwim using their live data. But these would be useless without Mathematica using polygon.io data where a static view of spreads slows down the process allowing me to orient myself relative to the insanity on ThinkOrSwim and TradingView. Nobody says building this stuff is easy but its very satisfying and has afforded some success. I attach an image only to show what is possible with MMA and to encourage perserveance.
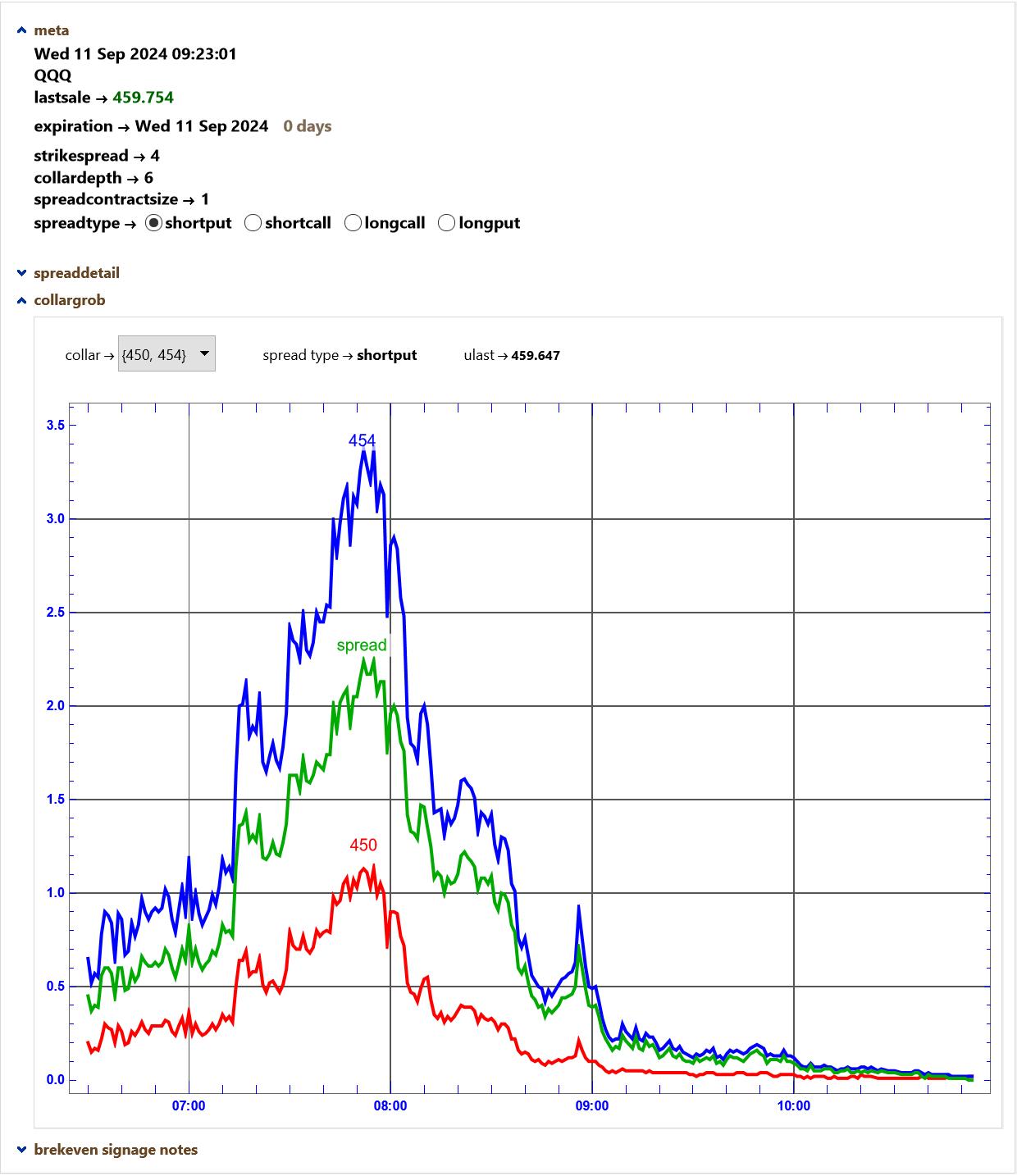