Eric,
You can take a look at the following. I think it gives a start on implementing the functionality that you desire. MMA has some nice Boolean logic functionality built in to make this easier.
First, I create a characteristic equation for the JK FF. Then, create a BooleanFunction
to implement the corresponding truth table:
jkFFEqn = (j \[And] \[Not] q) || (\[Not] k \[And] q)
jkFlipFlop = BooleanFunction[BooleanTable[jkFFEqn, {j, k, q}]]
Confirm that the correct truth table is generated:
TableForm[
Boole@BooleanTable[{j, k, q, jkFlipFlop[j, k, q]}, {j, k, q}],
TableHeadings -> {None, {j, k, q, "q[n+1]"}}]
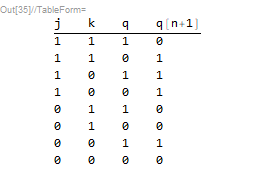
This function will generate the transition to the next state. It requires that the flip flop to be transitioned be initialized to a known state.
Note: I made some changes force the flip flop object state to be a Boolean value (True
, False
). This will make it unnecessary to convert (0, 1) values to (False, True) when chaining negated outputs to inputs.
Clear[jkFlipFlopNextState]
jkFlipFlopNextState[j_?(# == 0 || # == 1 &), k_?(# == 0 || # == 1 &),
flipflopObj_Symbol, id_Integer] :=
jkFlipFlopNextState[j != 0, k != 0, flipflopObj, id] /;
ValueQ[flipflopObj[id]]
jkFlipFlopNextState[j_?(# == 0 || # == 1 &),
k_?(# == False || # == True &), flipflopObj_Symbol, id_Integer] :=
jkFlipFlopNextState[j != 0, k, flipflopObj, id] /;
ValueQ[flipflopObj[id]]
jkFlipFlopNextState[j_?(# == False || # == True &),
k_?(# == 0 || # == 1 &), flipflopObj_Symbol, id_Integer] :=
jkFlipFlopNextState[j, k != 0, flipflopObj, id] /;
ValueQ[flipflopObj[id]]
jkFlipFlopNextState[j_?(# == False || # == True &),
k_?(# == False || # == True &), flipflopObj_Symbol,
id_Integer] := (flipflopObj[id] =
jkFlipFlop[j, k, flipflopObj[id]]) /; (flipflopObj[id] == False ||
flipflopObj[id] == True)
To test, pick a name for your JK FF and a unique integer ID and an initial output (Q) state. I choose False
as the initial state:
jkFF[1] = False
Then, run the state transition function. I generated some test input pairs (J, K) and mapped the transition function onto that list:
TableForm[{#[[1]], #[[2]], Boole@jkFF[1],
Boole@jkFlipFlopNextState[#[[1]], #[[2]],jkFF, 1]} & /@ Tuples[{0, 1}, 2],
TableHeadings -> {None, {j, k, q, "q[n+1]"}}]
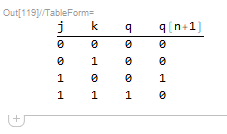
You can add more JK's simply by initializing them like I did for jkFF[1]
, Like this,
jkFF[2] = False (* This could be False or True *)
jkFF[3] = True (* etc, etc *)
Here's a JK FF configured as a toggler.
jkFF[1] = False;
Table[Boole@jkFlipFlopNextState[1,1, jkFF, 1], 10]
Out[125]:= {1, 0, 1, 0, 1, 0, 1, 0, 1, 0}
I didn't implement the clock since that seems to be a relatively simple extension to what I've done. If you desire, you can put all of this in a context (package) for localization. Hope this helps.