I'm sure some of this can be streamlined, but I haven't used these image processing functions much so I'm just doing what seems to make sense. I'm taking image
as the image you provided.
(* Let's get rid of the border *)
croppedImage = ImageCrop[image]
(* Let's slice off the left image. It will be our reference image. *)
leftImage = ImageTake[croppedImage, All, {1, Floor[ImageDimensions[croppedImage][[1]]/2] - 1}]
(* Let's slice off the right image. I'm taking a slightly smaller image to avoid edge artifacts. Maybe this isn't necessary. *)
rightImage = ImageTake[croppedImage, {2, -2}, {2 - Floor[ImageDimensions[croppedImage][[1]]/2], -2}]
(* Now we can use ImageAliign. Unfortunately, an edge artifact crops up, so we'll need to "erase" that later. *)
{alignedLeft, alignedRight} = ImageCrop[#, targetDims] & /@ ImageAlign[{leftImage, rightImage}, Background -> Black, TransformationClass -> "Translation"]
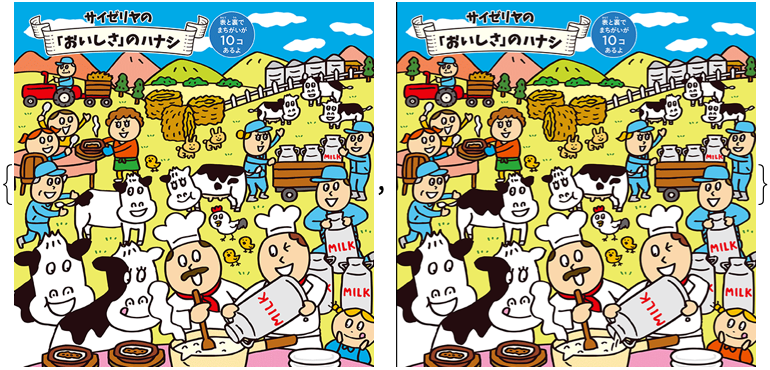
(* The edge artifact can be seen as a border. *)
borders = BorderDimensions[alignedRight]
(* {{1, 0}, {0, 0}} *)
(* Here we clean that up. I'm taking a bit extra, because I think there was also some anti-aliasing. *)
{cleanLeft, cleanRight} = ImagePad[#, -1 - borders] & /@ {alignedLeft, alignedRight}
(* We can see the diffs now. *)
diff = ImageDifference[cleanLeft, cleanRight]
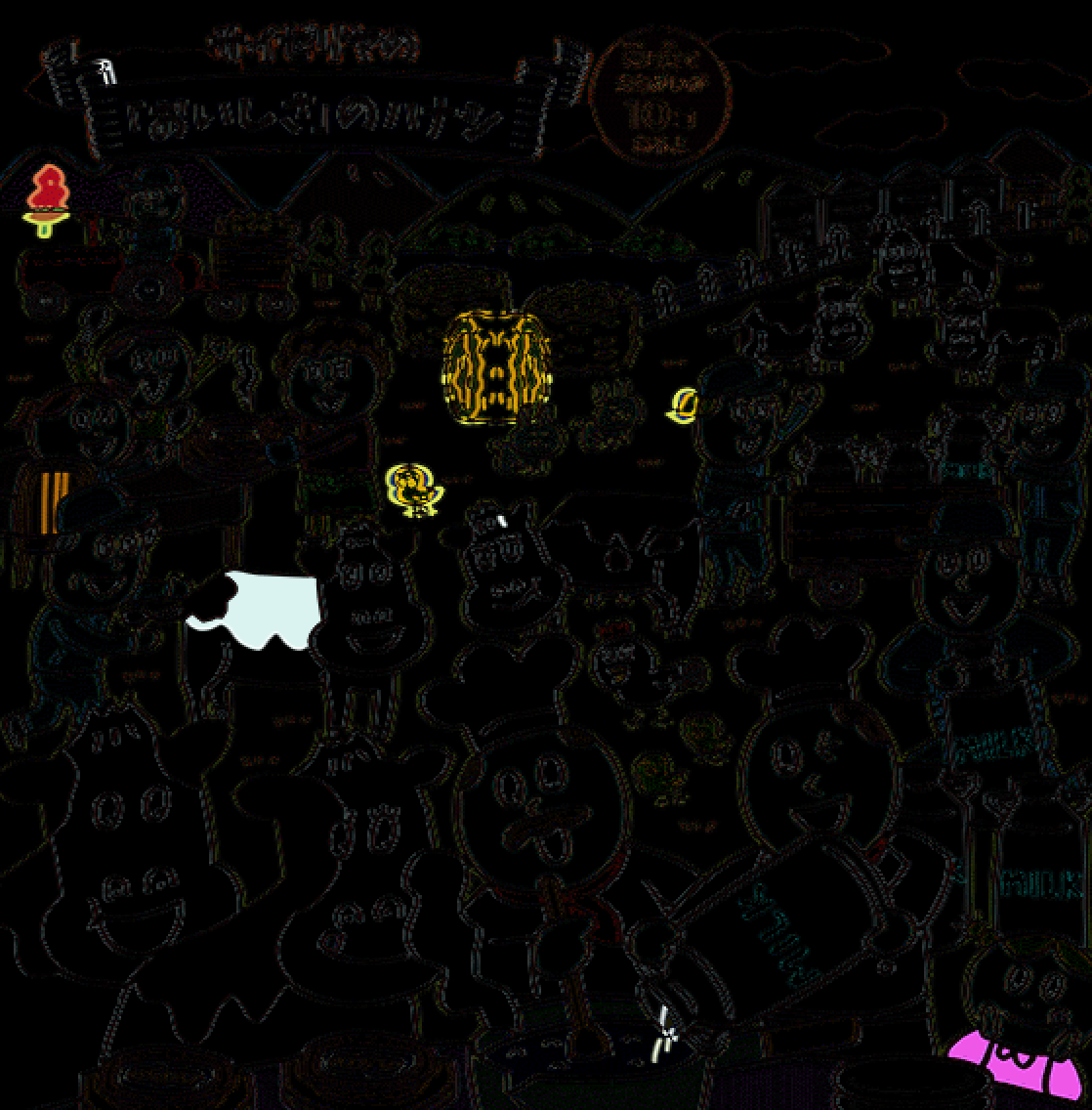
Unfortunately, the diff doesn't create nice solid components. The little details break things up into many small components. What I decided to do was to blur this diff, hoping that this will connect nearby details. I had to fiddle around to find a blurring radius that worked nicely. I also binarized it for accuracy. Here is what it looks like:
Blur[Binarize[diff, .4], 6]
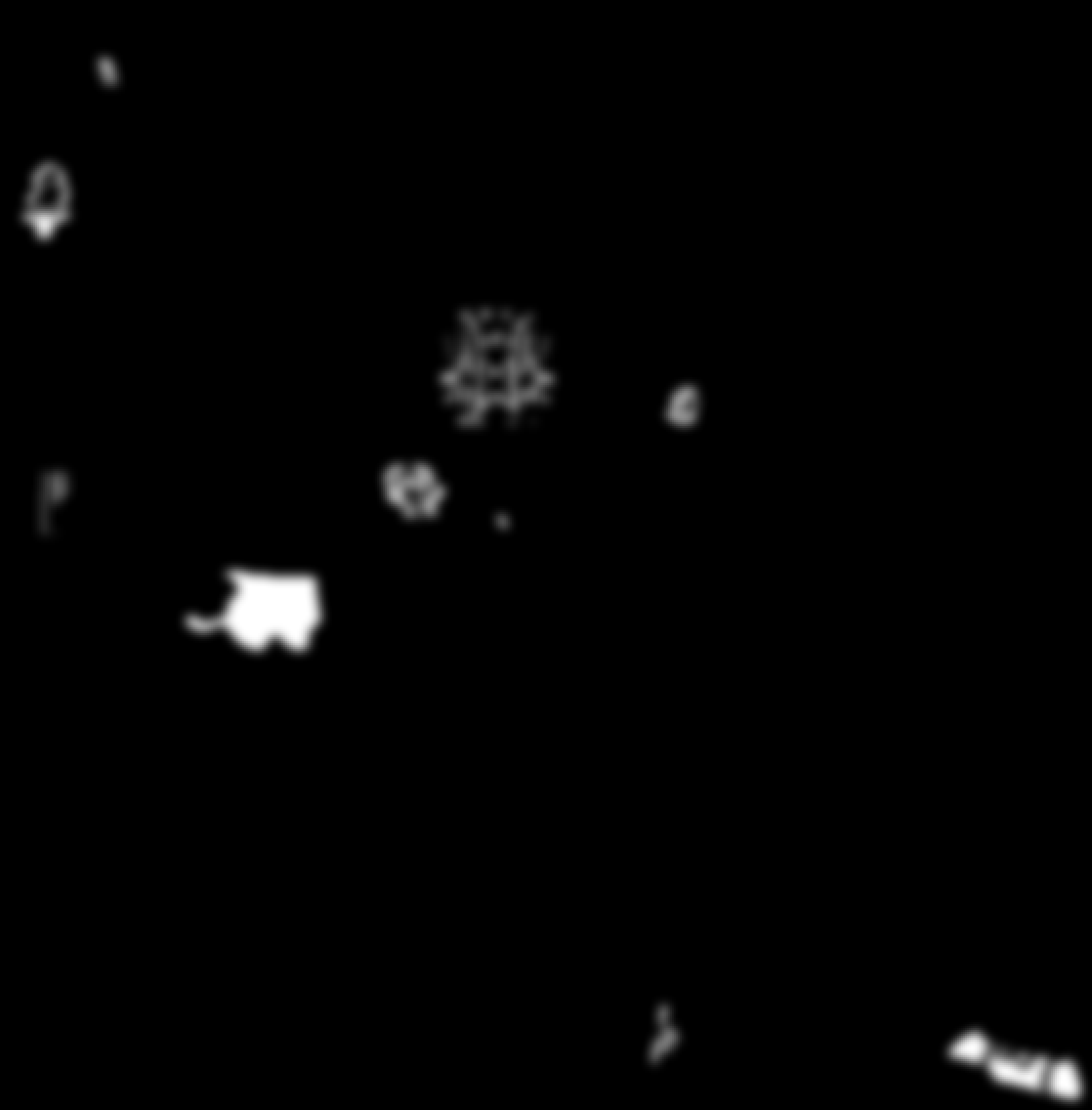
My plan now is to use MorphologicalComponents
. Here's what we can get now:
MorphologicalComponents[Blur[Binarize[diff, .4], 6], Method -> "BoundingDisk"] // Colorize
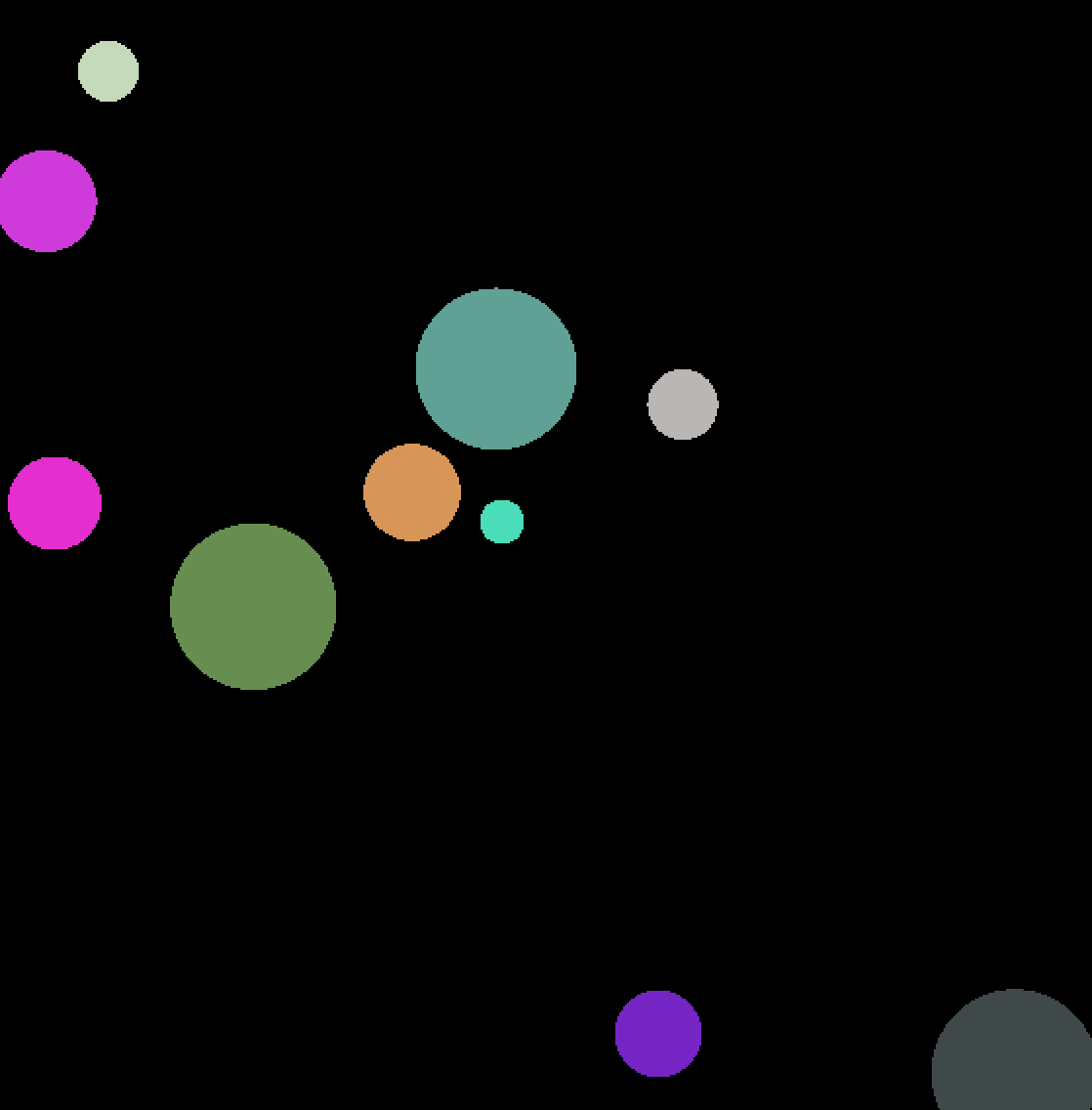
I'll combine the next few steps. We create perimeters, thicken them so they can be seen more easily, color them red for the same reason, and overlay this with our reference image.
diffOverlay =
ImageRecolor[
Colorize[
MorphologicalTransform[
MorphologicalPerimeter[MorphologicalComponents[Blur[Binarize[diff, .4], 6], Method -> "BoundingDisk"]],
Max]],
{Black -> Transparent, _ -> Red}]
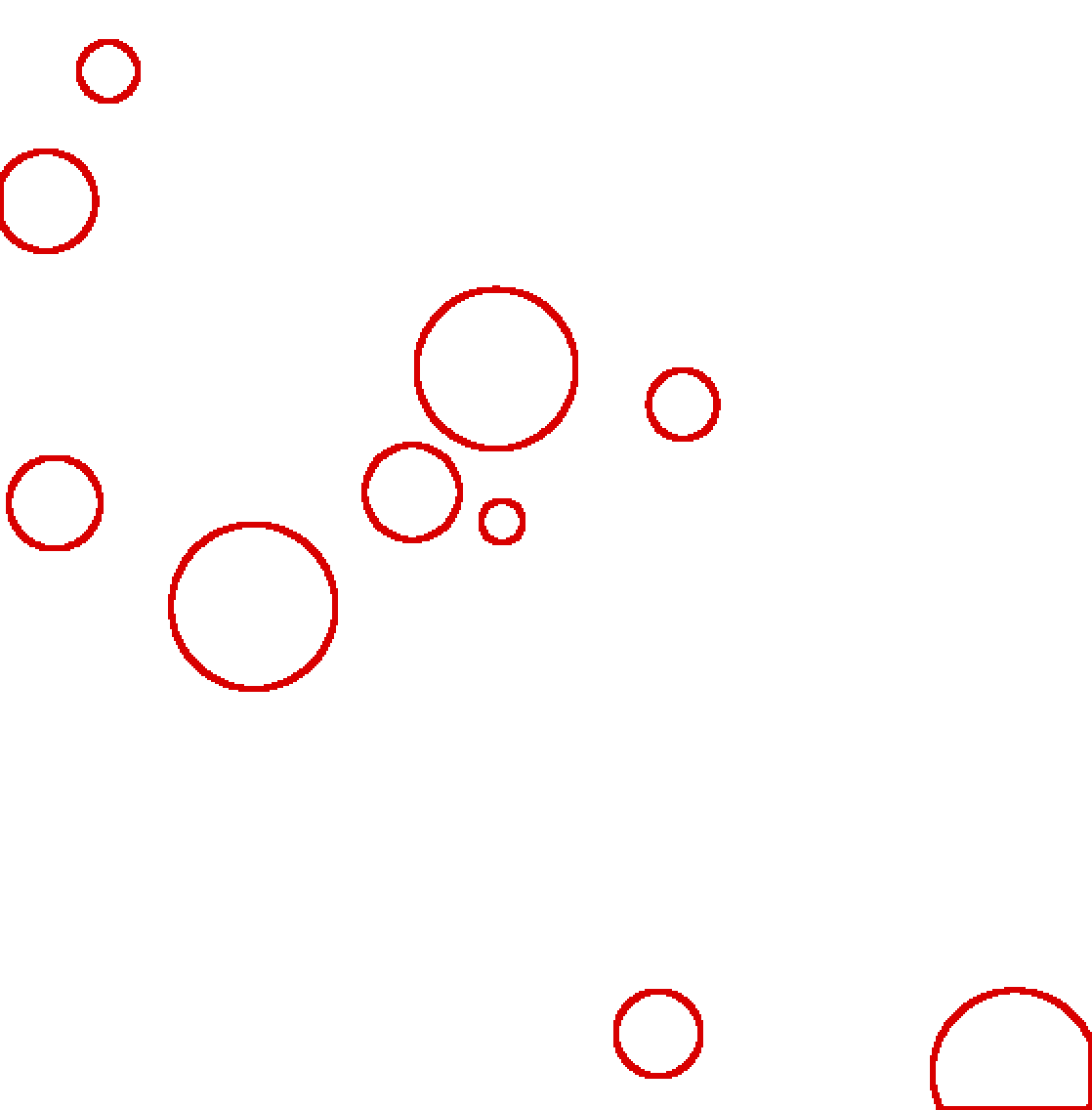
ImageCompose[cleanLeft, diffOverlay]
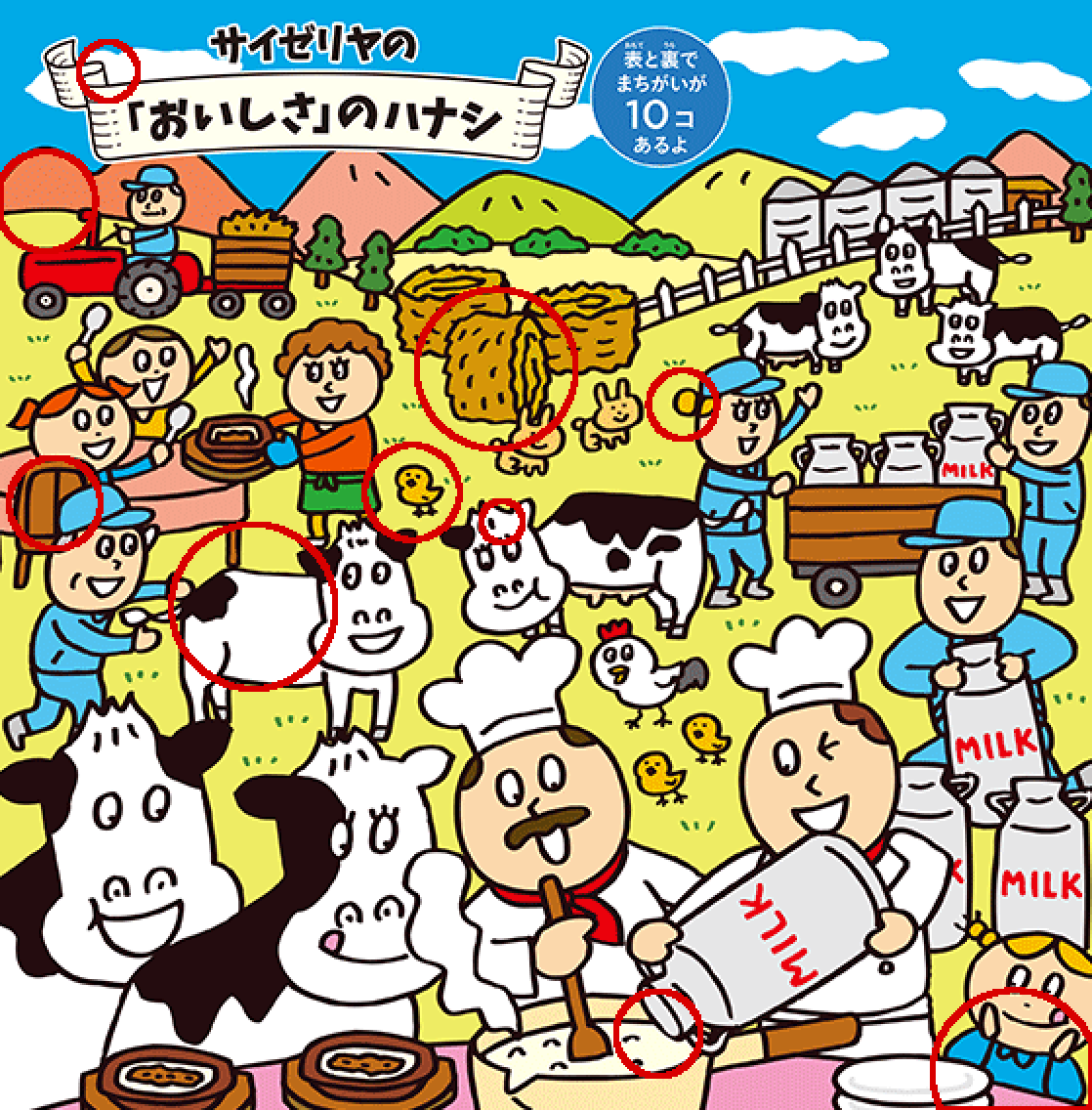