So what you need is to be able to read in from the Serial port as a stream, and from multiple ports concurrently? I will just say that this is still an "unsolved" problem in Mathematica with Serial ports, as this requires asynchronous updating of the dynamics, which doesn't really work well in my experiences. I think the best solution would be to have the Arduino spit out the values in some form of packet, something similar to the following:
\n a { 1 , 10 } \n b { 1 , 12 } \n c { 1 , 13 }
Where the \n (newline) delimiter will still allow you to split the values up by each reading, but then the 'a', 'b', 'c', etc. characters allow you to distinguish between which port the reading is coming from, so you could do something like the following:
RunScheduledTask[
(
rawdata = DeviceReadBuffer["Serial"];
individualPointsStringList = StringSplit[FromCharacterCode[rawdata], "\n"];
sortedPointsList = GatherBy[individualPointsStringList, StringTake[#, 1] &];
cleanFullPointsList = ToExpression /@ (StringDrop[#, 1] & /@ sortedPointsList);
AppendTo[sensorAPointsList,cleanFullPointsList[[1]]];
AppendTo[sensorBPointsList,cleanFullPointsList[[2]]];
)]
Etc. for all the sensors you have connected to your Arduino. This would make the variables sensorAPointsList, etc. have all the values you need and would be updated every one second. You can look at the documentation page for RunScheduledTask to change the timing if you want this to be updated sooner.
Now, with the following dynamic, we can set up a live plot of the sensor values with the following.
Dynamic[Row[{
ListLinePlot[sensorAPointsList,ImageSize->{250,250}],
ListLinePlot[sensorBPointsList,ImageSize->{250,250}]}]]
For me, this produced the following graphs:
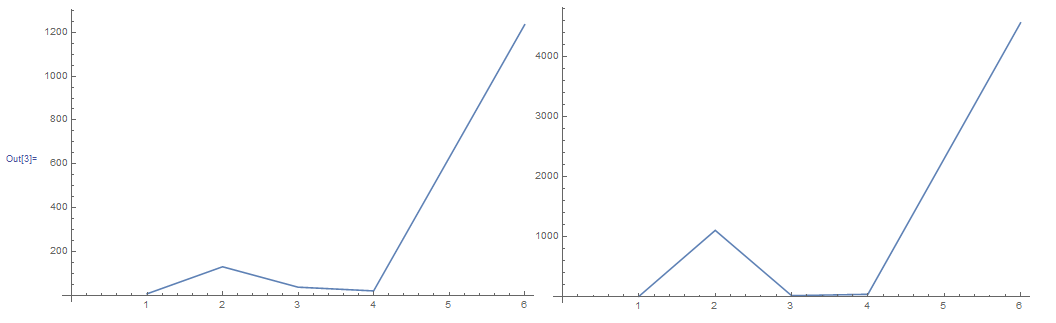