This post shows how to read an analog value from an Arduino Uno over a serial connection using the Wolfram Language.
To recreate this experiment you will need the following hardware:
As a first step upload the following sketch to the Arduino Uno. This sketch will contiously read the A0 analog
pin and send the data value (0-1023) over the serial connection; because we are writing single bytes we divide
the data value 'val' by 4 to rescale 0-1023 to 0-255:
int pin = 0;
int val = 0;
void setup() {
Serial.begin(115200);
}
void loop() {
val = analogRead(pin);
Serial.write(val/4);
delay(20);
}
Next configure your hardware as shown in the image below. Typically a potentiometer has three pins: one for voltage in, one for ground and one
pin which corresponds to the variable resistor.
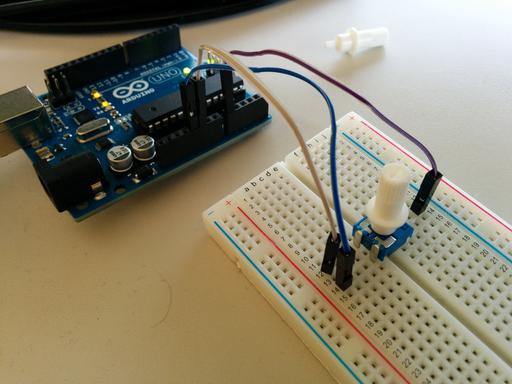
Next we need to connect Mathematica to the serial data link. This can be done with the following command:
serial = DeviceOpen["Serial", {"/dev/ttyACM0", "BaudRate" -> 115200}]
(When Mathematica 10 releases later this year, you can also run this experiment directly on a Windows or Mac computer, using for example the "COM" port on Windows).
Next we need to read the most current analog value.
ReadPotValue[] := Last[ DeviceReadBuffer[serial] ]
Now ReadPotValue[] will always return a current data value (like the number 157), so now we can read this value repeatedly using a ScheduledTask:
RunScheduledTask[val = ReadPotValue[], 0.33]
The 0.33 is the time interval here (3 times per second). On the Raspberry Pi this is close to the upper limit on how often you can read, especially when also running the next visualization step.
Finally you can visualize the current data value with an AngularGauge. Turning the potmeter connected to your Arduino will update the gauge in Mathematica:
AngularGauge[Dynamic[val], {0, 1023}]
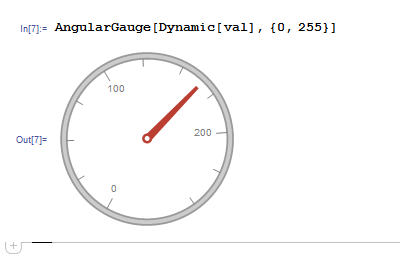
Here is a video showing everything in action:
http://www.youtube.com/watch?v=Ivzg5BY1GRw