This is very cool, thanks for sharing!
Here is a variation to transform a (black and white) image to a graphics expression, while taking into account multiple regions.
Throughout this post I will use the following test image:
image = Import["c:\\Users\\arnoudb.WRI\\paw.png"]
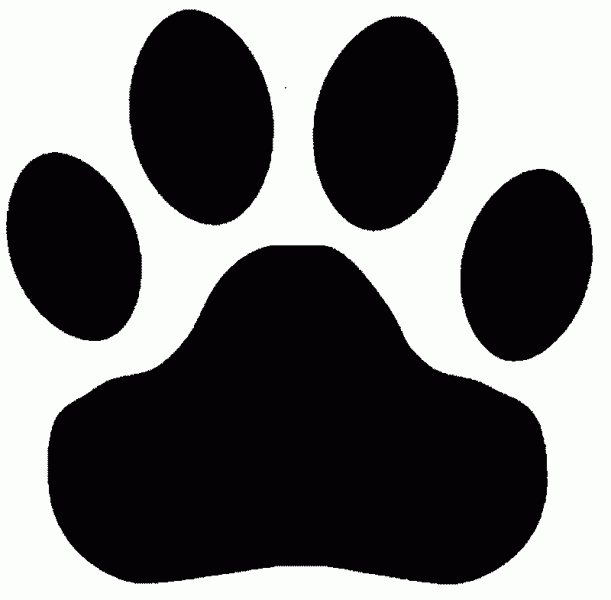
This is a helper function which uses MorphologicalComponents to split the image up into a list of images, each containing a single coherent part of the original image:
ImageToParts[image_] := Module[{components, max},
components = MorphologicalComponents[Binarize@ColorNegate[image]];
max = Max[Union[Flatten[components]]];
parts = Table[Image[components /. Table[i -> If[i == j, 1, 0], {i, max}]], {j, max}]
]
And here is an example of what it does (the third image is a tiny speck in the original):
ImageToParts[image]

This function uses the helper function to split up the original image and find the 'shortest tour' for the edge points of each sub-image:
ImageToGraphics[image_] := Module[
{dims, parts, edges, points, shortest, smooth, prims},
dims = ImageDimensions[image];
parts = ImageToParts[image];
prims = Table[
edges = EdgeDetect[part];
points = {Last[#], 1+Last[dims] - First[#]} & /@ N@Position[ImageData[edges], 1];
shortest = FindShortestTour[points][[2]];
smooth = Mean /@ Partition[points[[shortest]], 8, 1, {-1, 1}];
Polygon[smooth],
{part, parts}];
Graphics[
{EdgeForm[Black], Opacity[.5], Red, prims},
ImageSize -> dims,
PlotRange -> {{1, First[#]}, {1, Last[#]}} &[dims]
]
]
And here is the result, when calling it on the test image:
graphics = ImageToGraphics[image]
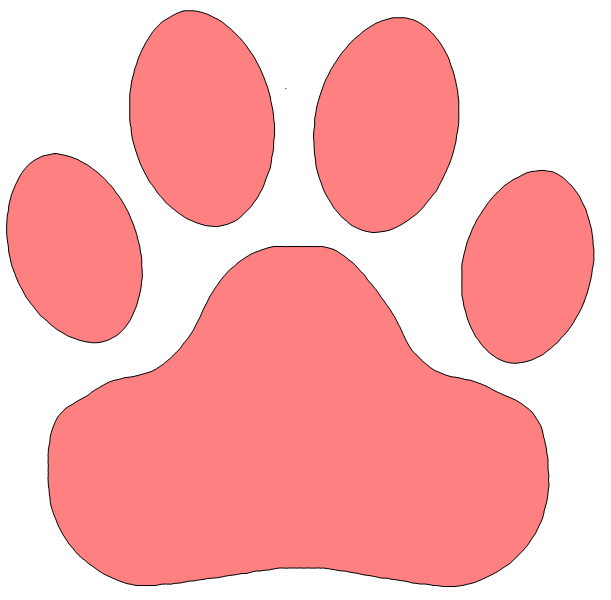
And this shows how the test image and the graphics expression match:
Overlay[{image, graphics}]
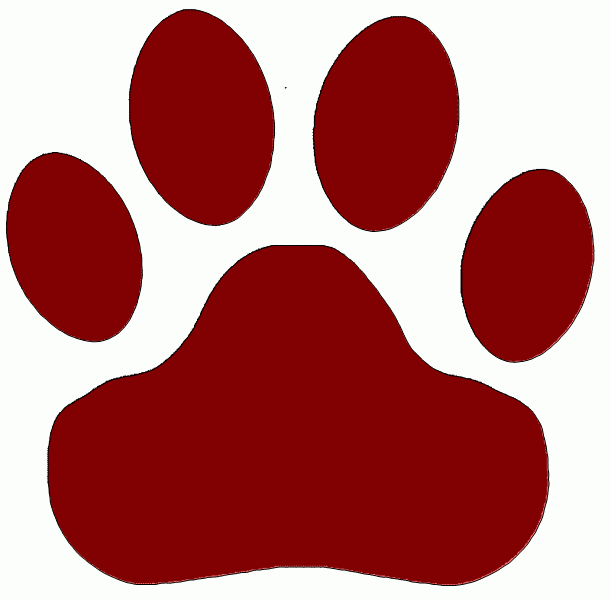