This is a continuation of a series of posts where I have illustrated how one can control a GSM module with the Wolfram Language.
In the two previous posts
Controlling a GSM module with the Wolfram Language
Using the Wolfram Language in a geo-location device
I explained how it is possible to send and receive SMS messages and find the remote location of a Sim908 GSM module attached to a R-Pi computer. The point of this post is to show how the Wolfram Language enables us to do similar tasks with a standard USB modem attached to any computer. Of course since a USB modem does not include a GPS, the geolocation is not possible but sending or receiving of SMS is possible and one can use the Wolfram Language to write programs to control computers remotely by sending them SMS messages.
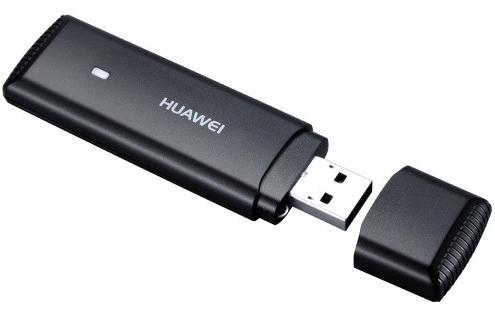
USB modems are not expensive and they are used to access high speed Internet networks with computers located in any place with cellular network coverage. A USB modem receives and sends its instructions by means of Hayes commands in a way explained in the above posts.
Here we need to adapt the code written for these cases to the present situation. The examples which we describe here were carried out in a Linux box but I expect that they can be carried over with computers running other operating systems. When a USB modem is hotplugged to a Linux computer a number of character devices are created. The precise devices can be found by executing the command dmesg
# dmesg
and examining its output. Sometimes it is necessary to carry out some previous configuration in order to have the modem detected, see for example here for some guidance. In this example the devices are "/dev/ttyUSB0" and "/dev/ttyUSB1" (in other cases there might be more or might have a different name). Next we need to find out which of these devices can be used to send the commands. Usually "/dev/ttyUSB0" can serve to this purpose but if this does not work for you try another one until you find a character device which works (in this post I shall use "/dev/ttyUSB0").
To be able to interact with the modem we create first channels to send Hayes commands and read their response
serialwrite = OpenWrite["/dev/ttyUSB0", BinaryFormat -> True]
serialread = DeviceOpen["Serial", {"/dev/ttyUSB0", "BaudRate" -> 115200}]
We use the stream serialwrite as the channel to send in the commands and the device object serialread to read the commands response. For example:
(* Send in command AT *)
BinaryWrite[serialwrite, "AT\r"];
(* Read the command response *)
FromCharacterCode@DeviceReadBuffer[serialread]
In this fashion we can adapt the code used in previous posts to the present situation. For example the following code will enable us to read and send SMS with the modem:
(* Send the PIN number of your SIM card *)
BinaryWrite[serialwrite, "AT+CPIN=****\r"];
(* If the PIN is correct the response should read OK *)
FromCharacterCode@DeviceReadBuffer[serialread]
(* Check that the modem is registered in the cellular network *)
BinaryWrite[serialwrite, "AT+COPS?\r"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Set modem in text mode *)
BinaryWrite[serialwrite, "AT+CMGF=1\r"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Select the SIM card memory to read the SMS messages *)
BinaryWrite[serialwrite, "AT+CPMS=\"SM\",\"SM\",\"SM\"\r"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Check the previous output and select a message to read. For example the second one *)
BinaryWrite[serialwrite, "AT+CMGR=1\r"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Now we send a SMS to a phone number *)
BinaryWrite[serialwrite, "AT+CMGS=\"123456789\"\r"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Insert SMS text *)
BinaryWrite[serialwrite, "Sent from my computer"];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Finish typing the SMS text *)
BinaryWrite[serialwrite, {26}];
FromCharacterCode@DeviceReadBuffer[serialread]
(* Wait till the recipient receives the SMS *)
We present next another Wolfram Language program which can be used as the starting point to control remotely a computer. This program checks every five seconds whether a new SMS has been received and when that happens sends a SMS acknowledging the reception. This last SMS is sent only if the incoming SMS text matches a predefined string.
(* Open serial port *)
serialread = DeviceOpen["Serial", {"/dev/ttyUSB0", "BaudRate" -> 115200}];
serialwrite = OpenWrite["/dev/ttyUSB0", BinaryFormat -> True];
(* Unlock SIM card *)
BinaryWrite[serialwrite, "AT+CPIN=****\r"];
(* Set SMS mode to text *)
BinaryWrite[serialwrite, "AT+CMGF=1\r"];
(* Flush serial port buffer (allow some time to process the previous set of instructions) *)
Pause[5];
DeviceReadBuffer[serialread];
(* Loop to query unread SMS messages *)
While[True,
(* Flush serial port buffer *)
DeviceReadBuffer[serialread];
(* Get unread SMS if any *)
BinaryWrite[serialwrite, "AT+CMGL=\"REC UNREAD\"\r"];
Pause[5];
message=FromCharacterCode@DeviceReadBuffer[serialread];
message=ImportString[message,"Table"];
Print[message];
message=Part[message,-3];
Print[message];
(* Check that sms text message matches a pre-defined string *)
If[message === {"password"},
(* Acknowledge reception of SMS *)
loc = "Message received";
(* Send confirmation via SMS to destination cell phone *)
BinaryWrite[serialwrite, "AT+CMGS=\"123456789\"\r"];
BinaryWrite[serialwrite, loc];
BinaryWrite[serialwrite, FromCharacterCode[{26}]];
Pause[10]
];
(* Flush serial port buffer *)
DeviceReadBuffer[serialread]
]
]
The program has to be run as a script. This is done by saving the code as ProgramName.m and then issuing in a shell terminal the command
# math -script ProgramName.m